Overview of Saving via Serialization
Often we need to save application data so that it can be reloaded when the application launches again and we can carry on where we left off.
When we have complex data structures (e.g. MutableLists of OOP objects), the simplest way to save the data within then to a file for later access, is to:
- Serialize the data into a text format such as JSON
- Write this JSON string to a text file (e.g. data.json)
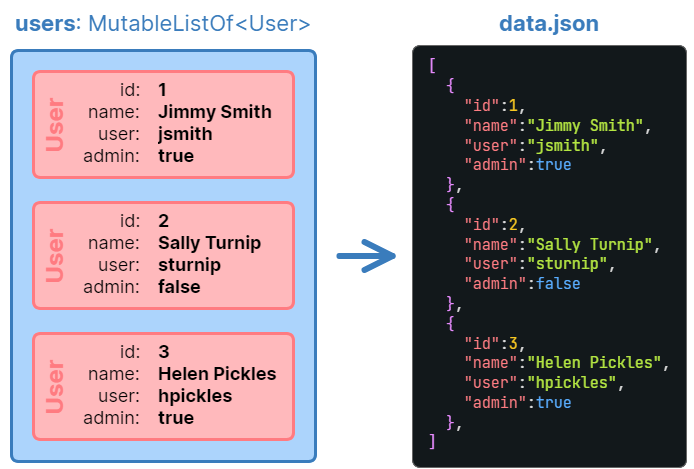